Simple Bash Script For File Managment on Apache Web Server
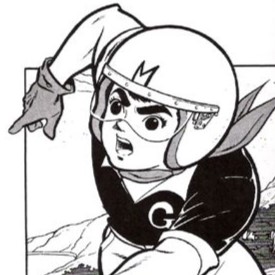
Author: Baden
May 5, 2023
Recently, I made this website you're looking at now. When creating this website, I learned that in order
to make my html visible to the outside world I needed a web server. I went with Apache just because I am
not familiar with it as much as IIS Servers (Window’s web server). I thought it would be a good thing to
push me out of my comfort zone.
When making an Apache Web Server you have to put your front-end assets (your HTML, CSS or images) in a
specific place, so when someone looks up your website’s domain, it can find the corresponding html to
display on the web browser. In this case Apache wants you to put assets in a folder called
/var/www/html. Simple enough, right? Well I found out pretty quickly this was not the case.
Because I am using an Ubuntu machine hosted by Google Cloud, I have to upload my files to the home
directory via SSH. That’s fine and good and all but with all of the files all piled up on the home
drive, I would have to manually use
sudo mv index.html /var/www/html
for each asset to get
it where I wanted it to be. Well, if you only have one or two assets, that’s fine, but I have loads of
assets! And I am a programmer, and we are a lazy people!
That is when I remembered a course at school I took called Linux Scripting. This course taught us the
basics of scripting on a Linux machine, using Bash, Korn Shell, and others. I knew I could make this job
a lot easier on my lazy mind by incorporating a script.
What is a script, you may be asking? A script can be compared to a movie script, but instead of actors
following the script your computer acts out the script. So that means the computer runs line by line
down the script doing exactly what is asked of it. So, I quickly opened up VS Code and whipped up a Bash
Script for my computer to follow. And it looked a little something like this:
#!/bin/bash
# move to the current directory
cd "$(dirname "$0")"
# loop through all files in the directory
for file in *; do
# check if the file is not the script itself and is a regular file
if [[ "$file" != "$(basename "$0")" && -f "$file" ]]; then
# check if the file has .png, .jpg, or .svg extension
if [[ "$file" =~ \.(png|jpg|svg)$ ]]; then
# move the file to /var/www/html/images
sudo mv "$file" /var/www/html/images/
else
# move the file to /var/www/html
sudo mv "$file" /var/www/html/
fi
fi
done
Don't get overwhelmed here, I know programming can look a little scary to the
untrained eye but look at it like a computer would, line by line.
This script is very simple, it grabs all of the files in the current directory, in this case, the home
directory. After which it then loops through the file to see if it not the script itself (I don’t want
the script to move itself, that would be bad lol) and then checks to see if the file is an image. If the
file is not an image it just goes straight to the /var/www/html directory and if it is an image it goes
to a different directory here: /var/www/html/images, just for the sake of my organization.
In conclusion, sometimes it pays to be lazy! This was definitely a learning point for me. I love being
able to find a problem or inconvenience and be able to solve it on my own with programming.